- Generate List Of Numbers
- C++ Random Number Code
- How To Generate Random Number In Dev C Download
- Generate Random Number C++ 11
- How To Generate Random Number In Dev C Online
- The C Standard Library
- C Standard Library Resources
Generate List Of Numbers
Jun 21, 2018 Here, we will implement a menu drive program in C to generate random password with various combinations of alphabets and special characters. Submitted by Hritik Raj, on June 21, 2018. Problem Statement: Write a menu driven program to generate password randomly. Constraint: password should consist of. C program to generate random numbers C program to generate pseudo-random numbers using rand and random function (Turbo C compiler only). As the random numbers are generated by an algorithm used in a function they are pseudo-random, this is the reason that word pseudo is used.
Random Number Generation C It is often useful to generate random numbers to produce simulations or games (or homework problems:) One way to generate these numbers in C. Jun 23, 2017 srand sets the seed which is used by rand to generate “random” numbers. If you don’t call srand before your first call to rand, it’s as if you had called srand(1) to set the seed to one. In short, srand — Set Seed for rand Function. This article is contributed by Shivam Pradhan. Generate a different random number each time, not the same one six times in a row. Use case scenario. I likened Predictability's problem to a bag of six bits of paper, each with a value from 0 to 5 written on it. A piece of paper is drawn from the bag each time a new value is required. If the bag is empty, then the numbers are put back into the bag. Feb 21, 2008 Dev-C; Discussion; Dev-C. Dev C random number generator Forum: Bloodshed Software Forum. Creator: Mathew. I got the proram to do the design for me. Now its just that I am planning ot generate a random number between 0 and 8 so that when the user click a button, it will generate a number and will put a cross on that panel. C program to generate random numbers. Randomize – this function is used to generate random numbers each time, when you run program. Rand – this function is used to return random number from 0 to RANDMAX-1. Here RANDMAX is the maximum range of the number. If you want to generate random numbers from 0 to 99 then RANDMAX will be 100.
- C Programming Resources
- Selected Reading
Description
The C library function int rand(void) returns a pseudo-random number in the range of 0 to RAND_MAX.
RAND_MAX is a constant whose default value may vary between implementations but it is granted to be at least 32767.
Declaration
Following is the declaration for rand() function.
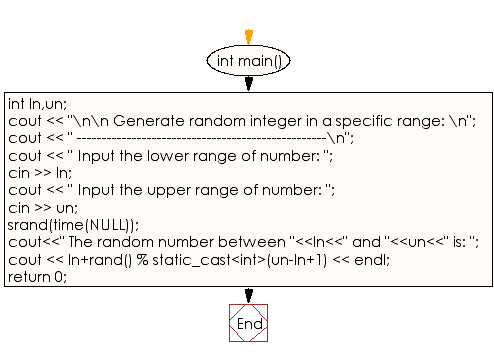
Parameters
NA
Return Value

This function returns an integer value between 0 and RAND_MAX.
Example
The following example shows the usage of rand() function.
Let us compile and run the above program that will produce the following result −
C++ Random Number Code
Random number generators fulfill a number of purposes. Everything from games to simulations require a random number generator to work properly. Randomness finds its way into business what-if scenarios as well. In short, you need to add random output to your application in many situations.
Creating a random number isn’t hard. All you need to do is call a random number function as shown in the RandomNumberGenerator example:
Actually, not one of the random number generators in the Standard Library works properly — imagine that! They are all pseudorandom number generators: The numbers are distributed such that it appears that you see a random sequence, but given enough time and patience, eventually the sequence repeats.
How To Generate Random Number In Dev C Download
In fact, if you don’t set a seed value for your random number generator, you can obtain predictable sequences of numbers every time. How boring. Here is typical output from this example:
Generate Random Number C++ 11
The first line of code in main() sets the seed by using the system time. Using the system time ensures a certain level of randomness in the starting value — and therefore a level of randomness for your application as a whole. If you comment out this line of code, you see the same output every time you run the application.
The example application uses rand() to create the random value. When you take the modulus of the random number, you obtain an output that is within a specific range — 12 in this case. The example ends by adding 1 to the random number because there isn’t any month 0 in the calendar, and then outputs the month number for you.
The Standard Library provides access to two types of pseudorandom number generators. The first type requires that you set a seed value. The second type requires that you provide an input value with each call and doesn’t require a seed value. Each generator outputs a different data type, so you can choose the kind of random number you obtain.
The table lists the random number generators and tells you what data type they output.
Function | Output Type | Seed Required? |
---|---|---|
rand | integer | yes |
drand48 | double | yes |
erand48 | double | no |
lrand48 | long | yes |
nrand48 | long | no |
mrand48 | signed long | yes |
jrand48 | signed long | no |
Now that you know about the pseudorandom number generators, look at the seed functions used to prime them. The following table lists the seed functions and their associated pseudorandom number generator functions.
How To Generate Random Number In Dev C Online
Function | Associated Pseudorandom Number Generator Function |
---|---|
srand | rand |
srand48 | drand48 |
seed48 | mrand48 |
lcong48 | lrand48 |