For example, when you are displaying number from 1 to 100 you may want set the value of a variable to 1 and display it 100 times, increasing its value by 1 on each loop iteration. In C we have three types of basic loops: for, while and do-while. In this tutorial we will learn how to use “for loop” in C. From C All-in-One For Dummies, 3rd Edition. By John Paul Mueller, Jeff Cogswell. C is a popular programming language because it’s powerful, fast, easy to use, standardized, and more. Whether you are new to C programming or an advanced user, check out the following information on C mistakes, header files, and syntax. C language is a direct descendant of C programming language with additional features such as type checking, object oriented programming, exception handling etc. You can call it a “better C”. It was developed by Bjarne Stroustrup.
A loop is used for executing a block of statements repeatedly until a particular condition is satisfied. For example, when you are displaying number from 1 to 100 you may want set the value of a variable to 1 and display it 100 times, increasing its value by 1 on each loop iteration.
In C++ we have three types of basic loops: for, while and do-while. In this tutorial we will learn how to use “for loop” in C++.
C String and String functions with examples: String is an array of characters. Learn how to use strings in C programming along with string functions. Nov 29, 2016 Hansoft is the agile project management tool for enterprise teams. Fast, efficient, and flexible, Hansoft empowers teams to collaborate more efficiently so they can advance together and build better products. Hansoft runs natively on leading operating sytems including OS. Format Source Code within Dev-C. The following instructions allow you to format source code files in Dev-C using FormatCode command line tool. Add a Tool Menu that will invoke FormatCode. Click 'Tools' - 'Configure Tools.' Click the 'Add' button to add a new tool.
Syntax of for loop
Flow of Execution of the for Loop
As a program executes, the interpreter always keeps track of which statement is about to be executed. We call this the control flow, or the flow of execution of the program.
First step: In for loop, initialization happens first and only once, which means that the initialization part of for loop only executes once.
Second step: Condition in for loop is evaluated on each loop iteration, if the condition is true then the statements inside for for loop body gets executed. Once the condition returns false, the statements in for loop does not execute and the control gets transferred to the next statement in the program after for loop.
Third step: After every execution of for loop’s body, the increment/decrement part of for loop executes that updates the loop counter.

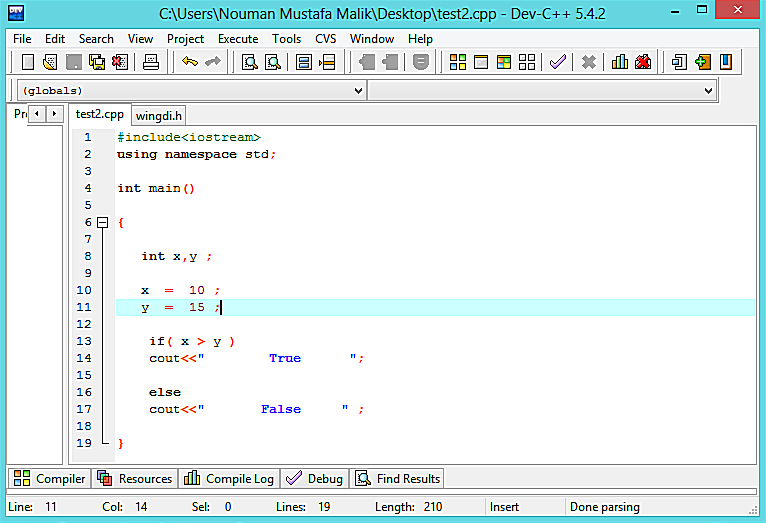
Fourth step: After third step, the control jumps to second step and condition is re-evaluated.
The steps from second to fourth repeats until the loop condition returns false.
Example of a Simple For loop in C++
Here in the loop initialization part I have set the value of variable i to 1, condition is i<=6 and on each loop iteration the value of i increments by 1.
Output:
Infinite for loop in C++
C++ Syntax Guide
A loop is said to be infinite when it executes repeatedly and never stops. This usually happens by mistake. When you set the condition in for loop in such a way that it never return false, it becomes infinite loop.
For example:
This is an infinite loop as we are incrementing the value of i so it would always satisfy the condition i>=1, the condition would never return false.
Basic Syntax Of Dev C Language
Here is another example of infinite for loop:
C++ Syntax Pdf
Example: display elements of array using for loop
Basic Syntax Of Dev C Example
Output: